Industry-Leading
Time Series Database
For the IoT
Our mission is to revolutionize the field of big data by developing cutting-edge software solutions that empower enterprises to utilize the full potential of time-series data. From seamless data collection and storage to efficient querying, powerful analysis, and meaningful application, we strive to enable enterprises to extract maximum value from their data while minimizing costs.
Programming Example
Select your preferred programming language to view the corresponding source code
package org.apache.iotdb;
import org.apache.iotdb.isession.SessionDataSet;
import org.apache.iotdb.rpc.IoTDBConnectionException;
import org.apache.iotdb.rpc.StatementExecutionException;
import org.apache.iotdb.session.Session;
import org.apache.iotdb.tsfile.write.record.Tablet;
import org.apache.iotdb.tsfile.write.schema.MeasurementSchema;
import java.util.ArrayList;
import java.util.List;
public class SessionExample {
private static Session session;
public static void main(String[] args)
throws IoTDBConnectionException, StatementExecutionException {
session =
new Session.Builder()
.host("172.0.0.1")
.port(6667)
.username("root")
.password("root")
.build();
session.open(false);
List<MeasurementSchema> schemaList = new ArrayList<>();
schemaList.add(new MeasurementSchema("s1", TSDataType.FLOAT));
schemaList.add(new MeasurementSchema("s2", TSDataType.FLOAT));
schemaList.add(new MeasurementSchema("s3", TSDataType.FLOAT));
Tablet tablet = new Tablet("root.db.d1", schemaList, 10);
tablet.addTimestamp(0, 1);
tablet.addValue("s1", 0, 1.23f);
tablet.addValue("s2", 0, 1.23f);
tablet.addValue("s3", 0, 1.23f);
tablet.rowSize++;
session.insertTablet(tablet);
tablet.reset();
try (SessionDataSet dataSet = session.executeQueryStatement("select ** from root.db")) {
while (dataSet.hasNext()) {
System.out.println(dataSet.next());
}
}
session.close();
}
}
from iotdb.Session import Session
from iotdb.utils.IoTDBConstants import TSDataType
from iotdb.utils.Tablet import Tablet
ip = "127.0.0.1"
port = "6667"
username = "root"
password = "root"
session = Session(ip, port, username, password)
session.open(False)
measurements = ["s_01", "s_02", "s_03", "s_04", "s_05", "s_06"]
data_types = [
TSDataType.BOOLEAN,
TSDataType.INT32,
TSDataType.INT64,
TSDataType.FLOAT,
TSDataType.DOUBLE,
TSDataType.TEXT,
]
values = [
[False, 10, 11, 1.1, 10011.1, "test01"],
[True, 100, 11111, 1.25, 101.0, "test02"],
[False, 100, 1, 188.1, 688.25, "test03"],
[True, 0, 0, 0, 6.25, "test04"],
]
timestamps = [1, 2, 3, 4]
tablet = Tablet(
"root.db.d_03", measurements, data_types, values, timestamps
)
session.insert_tablet(tablet)
with session.execute_statement(
"select ** from root.db"
) as session_data_set:
while session_data_set.has_next():
print(session_data_set.next())
session.close()
#include "Session.h"
#include <iostream>
#include <string>
#include <vector>
#include <sstream>
int main(int argc, char **argv) {
Session *session = new Session("127.0.0.1", 6667, "root", "root");
session->open();
std::vector<std::pair<std::string, TSDataType::TSDataType>> schemas;
schemas.push_back({"s0", TSDataType::INT64});
schemas.push_back({"s1", TSDataType::INT64});
schemas.push_back({"s2", TSDataType::INT64});
int64_t val = 0;
Tablet tablet("root.db.d1", schemas, /*maxRowNum=*/ 10);
tablet.rowSize++;
tablet.timestamps[0] = 0;
val=100; tablet.addValue(/*schemaId=*/ 0, /*rowIndex=*/ 0, /*valAddr=*/ &val);
val=200; tablet.addValue(/*schemaId=*/ 1, /*rowIndex=*/ 0, /*valAddr=*/ &val);
val=300; tablet.addValue(/*schemaId=*/ 2, /*rowIndex=*/ 0, /*valAddr=*/ &val);
session->insertTablet(tablet);
tablet.reset();
std::unique_ptr<SessionDataSet> res = session->executeQueryStatement("select ** from root.db");
while (res->hasNext()) {
std::cout << res->next()->toString() << std::endl;
}
res.reset();
session->close();
delete session;
return 0;
}
package main
import (
"fmt"
"log"
"github.com/apache/iotdb-client-go/client"
)
func main() {
config := &client.Config{
Host: "127.0.0.1",
Port: "6667",
UserName: "root",
Password: "root",
}
session := client.NewSession(config)
if err := session.Open(false, 0); err != nil {
log.Fatal(err)
}
defer session.Close() // close session at end of main()
rowCount := 3
tablet, err := client.NewTablet("root.db.d1", []*client.MeasurementSchema{
{
Measurement: "restart_count",
DataType: client.INT32,
Encoding: client.RLE,
Compressor: client.SNAPPY,
}, {
Measurement: "price",
DataType: client.DOUBLE,
Encoding: client.GORILLA,
Compressor: client.SNAPPY,
}, {
Measurement: "description",
DataType: client.TEXT,
Encoding: client.PLAIN,
Compressor: client.SNAPPY,
},
}, rowCount)
if err != nil {
fmt.Errorf("Tablet create error:", err)
return
}
timestampList := []int64{0, 1, 2}
valuesInt32List := []int32{5, -99999, 123456}
valuesDoubleList := []float64{-0.001, 10e5, 54321.0}
valuesTextList := []string{"test1", "test2", "test3"}
for row := 0; row < rowCount; row++ {
tablet.SetTimestamp(timestampList[row], row)
tablet.SetValueAt(valuesInt32List[row], 0, row)
tablet.SetValueAt(valuesDoubleList[row], 1, row)
tablet.SetValueAt(valuesTextList[row], 2, row)
}
session.InsertTablet(tablet, false)
var timeoutInMs int64
timeoutInMs = 1000
sql := "select ** from root.db"
dataset, err := session.ExecuteQueryStatement(sql, &timeoutInMs)
defer dataset.Close()
if err == nil {
for next, err := dataset.Next(); err == nil && next; next, err = dataset.Next() {
record, _ := dataset.GetRowRecord()
fields := record.GetFields()
for _, field := range fields {
fmt.Print(field.GetValue(), "\t")
}
fmt.Println()
}
} else {
log.Println(err)
}
}
Java
Python
C++
Go
-
Tens of Millions pts/s
Supports ingesting tens of millions of points per second on each node
-
100:1 Compression
Achieves over 10:1 lossless and up to 100:1 lossy compression
-
Millisecond Queries
Enables millisecond-level query response for terabyte-scale data
-
Billions of Tags
Handles tens of thousands of tags per device and billions across devices
Best Practices in the Industry
Addressing scenarios ranging from thousands to billions of data points
Celebrated by Academia and Industry
Drawing global contributors to co-create an open-source community
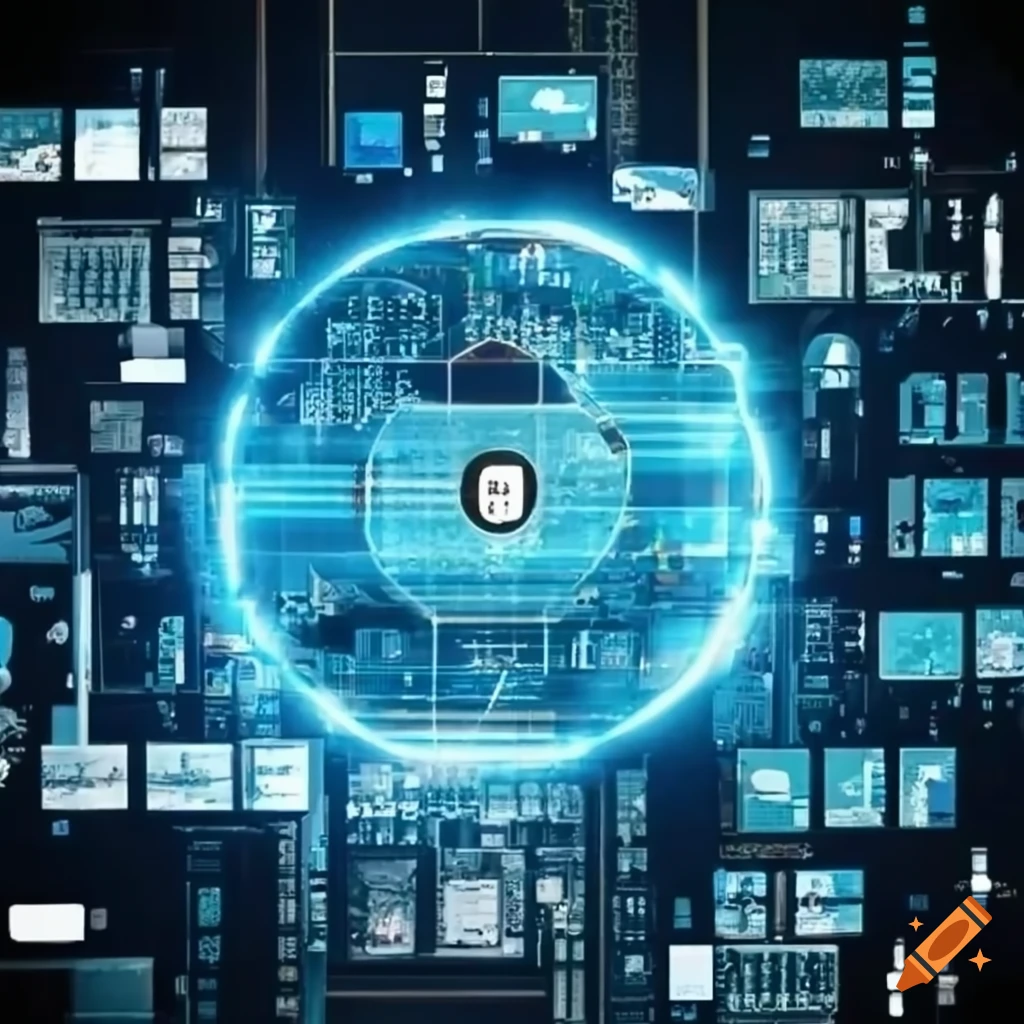
Optimizing (I)IoT Performance: The Apache IoTDB Solution for Data Challenges
Apache IoTDB is a fascinating project, solving many complex problems easily by simple design choices. However, this flexibility does not come with hidden costs with respect to performance or complexity.
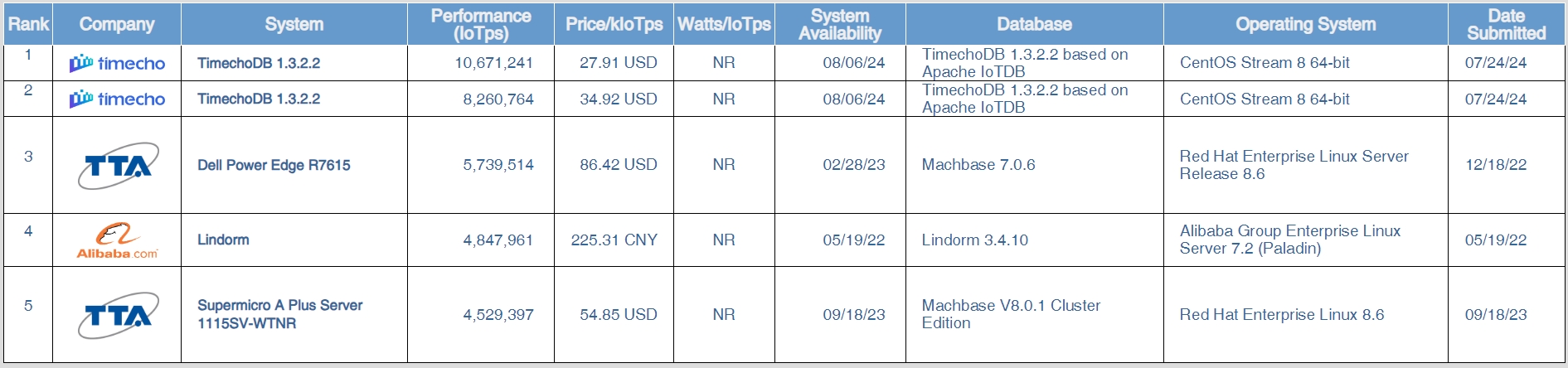
Apache IoTDB at the Core: New Records in TPCx-IoT Benchmarking
2024-09-10On August 6th, 2024, the Transaction Processing Performance Council (TPC) released TPCx-IoT benchmark results, highlighting TimechoDB (based on Apache
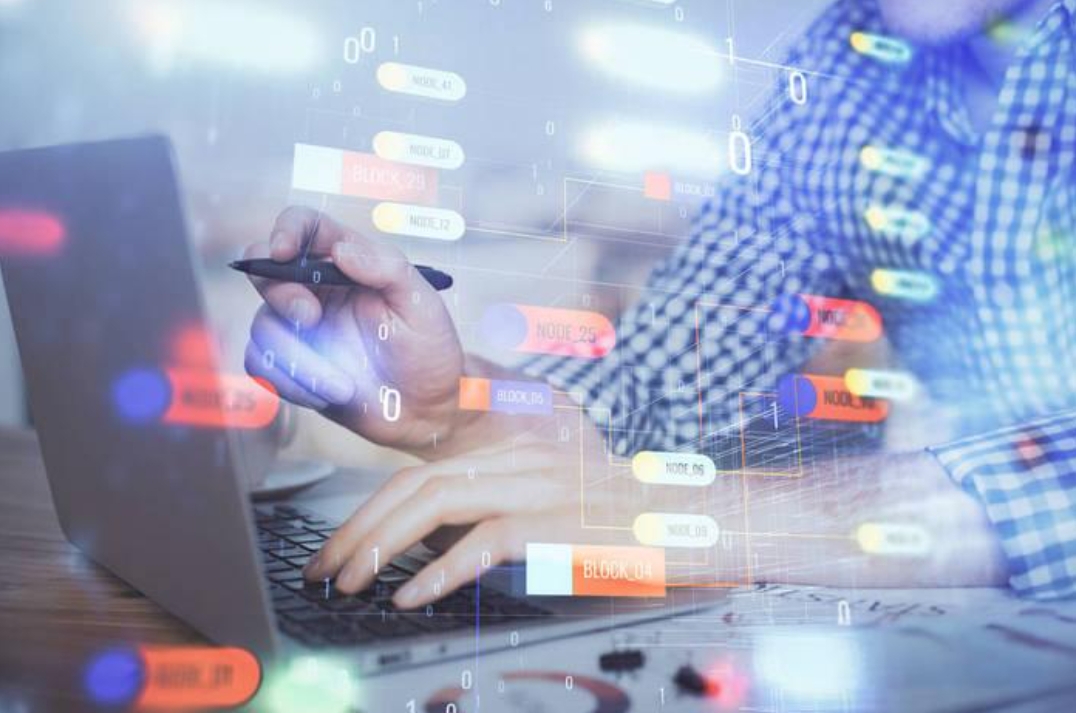
Three Different Deployment Forms of Apache IoTDB
2024-08-22Currently, Apache IoTDB supports three deployment forms—standalone deployment, cluster deployment, and dual-active deployment. This article will revea
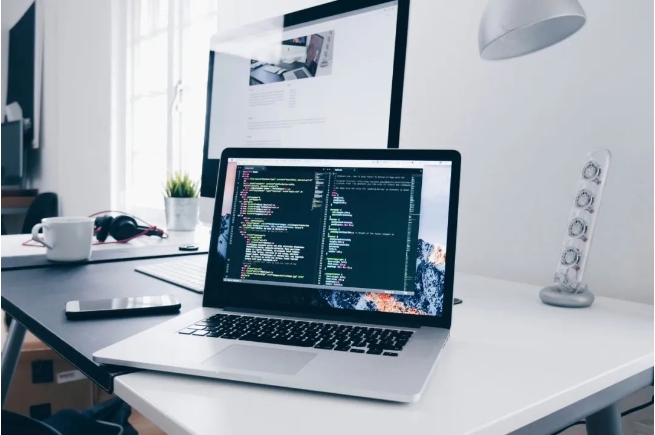
Mastering Memory Settings for Apache IoTDB Clusters
2024-08-15Apache IoTDB is a comprehensive data management engine designed for the collection, storage, and analysis of time series data. The performance and sta